我们可以通过JPQL来代替JPA的命名规则
下面先来一个简单的Demo
简单的JPQL例子
1.1、创建Theme的model
package com.fcors.fcors.model;
import lombok.Getter;
import lombok.Setter;
import javax.persistence.*;
import java.sql.Timestamp;
import java.util.Objects;
@Entity
@Getter
@Setter
@Table(name = "theme", schema = "missyou", catalog = "")
public class ThemeEntity extends BaseEntity{
@Id
@Column(name = "id")
private Long id;
private String title;
private String description;
private String name;
private String tplName;
private String entranceImg;
private String extend;
private String internalTopImg;
private String titleImg;
private Boolean online;
}
1.2、创建JPQL的Repository
package com.fcors.fcors.repository;
import com.fcors.fcors.model.ThemeEntity;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Query;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public interface ThemeRepository extends JpaRepository<ThemeEntity,Long> {
@Query("select t from ThemeEntity t where t.name in (:names)")
List<ThemeEntity> findByNames(List<String> names);
}
1.3、创建Services
接口:ThemeService
package com.fcors.fcors.service;
import com.fcors.fcors.model.ThemeEntity;
import java.util.List;
public interface ThemeService {
List<ThemeEntity> findByNames(List<String> names);
}
方法类:ThemeServiceImpl
package com.fcors.fcors.service;
import com.fcors.fcors.model.ThemeEntity;
import com.fcors.fcors.repository.ThemeRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class ThemeServiceImpl implements ThemeService{
@Autowired
private ThemeRepository themeRepository;
public List<ThemeEntity> findByNames(List<String> names){
return themeRepository.findByNames(names);
}
}
1.4、创建Controller
package com.fcors.fcors.api.sample.v1;
import com.fcors.fcors.model.ThemeEntity;
import com.fcors.fcors.service.ThemeService;
import com.github.dozermapper.core.DozerBeanMapperBuilder;
import com.github.dozermapper.core.Mapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.Arrays;
import java.util.List;
@RestController
@RequestMapping("theme")
@Validated
public class ThemeController {
@Autowired
private ThemeService themeService;
@GetMapping("/by/names")
public List<ThemeEntity> getThemeGroupByNames(@RequestParam(name = "names") String names) {
List<String> nameList = Arrays.asList(names.split(","));
List<ThemeEntity> themes = themeService.findByNames(nameList);
return themes;
}
}
1.5测试结果
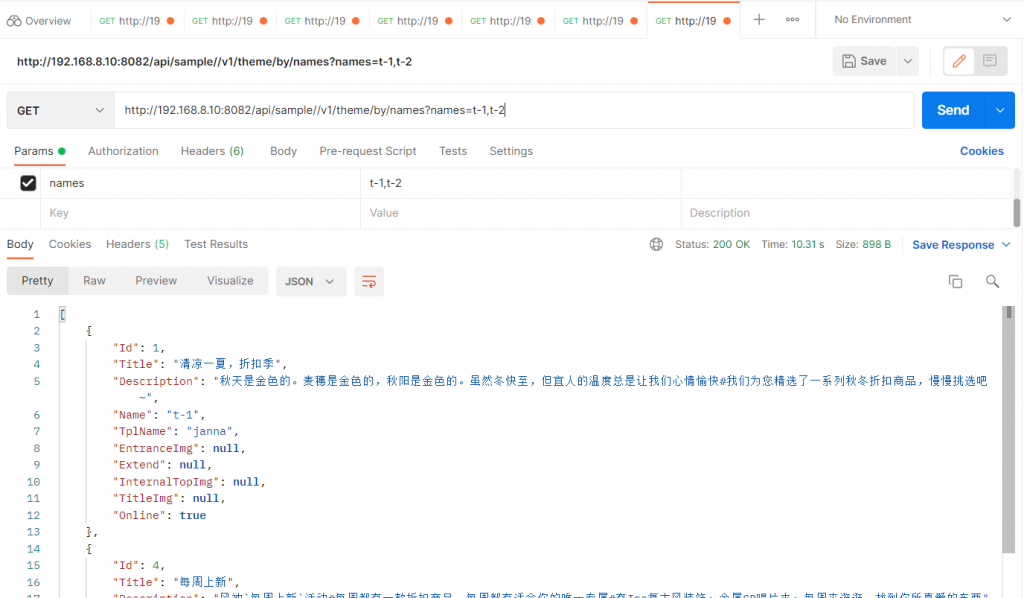